Simple Java Program
On my journey to get back to coding on a more regular basis. I decided to do a Java programming exercise where I can call different methods in a program. What better way than to practice with a classic program to calculate simple math problems. The project requirements are the following:
1- Collect two numbers from the user and store them in variables.
2- The program shall allow the user to add, subtract, multiply and divide.
3- Allow the user to choose what math operation he/she wants to use.
4- In addition, since I will be collecting input from a user we will need to validate the data from the user.
5- Finally provide the result at the end of the application.
We shall call it SimpleCalculator
First step in the program give it a name and assign the public class the name of the Java application. I called my application SimpleCalculator.
public class SimpleCalculator {
public static void main(String[] args){
}
}
Declare the variables
This application will comprise of primarily four important things. The numbers entered by the user, the operator used in order to determine how we will calculate, and finally the result. So since we don’t want to limit the user to only entering integers we should set our number and result variables to double. In addition we need to initialize to 0. The operator while its only one character that we will need I don’t want to parse the data entered, so we set the operator to a String data type.
public class SimpleCalculator {
public static void main(String[] args){
double num1 = 0, num2 = 0, result = 0;
String operator;
}
}
Scanner Class
In order to get input from the user we will need to use the Scanner Class. The Scanner Class is a class in the java.util package and its used for obtaining the input of the primitive data types like int, double, etc. and strings. To use this class we will need to import the java package. We do this by adding at the top of the code, before the public class statement. To create an object of Scanner class, we usually pass the predefined object System.in, which represents the standard input stream. The scanner object will be called input.
import java.util.Scanner;
public class SimpleCalculator {
public static void main(String[] args){
double num1 = 0, num2 = 0, result = 0;
String operator;
/* Scanner class is used to collect user input */
Scanner input = new Scanner (System.in);
Collect the data
Ok, so now we can output the request to the user to enter the numbers, and then scan the input stream into the variables. We can use the nextDouble() method in order to store the data entered into the defined double variables. After requesting the numbers we need to request what operation the user wants to use to conduct the calculation. Since we will store in a String, we can use the next() method in order to collect the operator.
System.out.print("Number 1: ");
num1 = input.nextDouble();
System.out.print("Number 2: ");
num2 = input.nextDouble();
System.out.print("+, -, *, / Operator? ");
operator = input.next();
Let’s create our arithmetic methods
The methods will be rather simple. We will just send two parameters which are the numbers entered by the user. Then return back those numbers using the corresponding arithmetic. For example to add the numbers we use a created method called addNumbers. We shall also send the two parameters: num1, num2. The method will return a double value and we can store the result of the operation in the double variable named result. This should only be executed if the user entered the “+”.
if(operator.equals("+")){
result = addNumbers(num1, num2);
}
}
static double addNumbers(double num1, double num2) {
return num1 + num2;
}
}
Repeat the process
Now we just repeat the process with the other operators. We shall call them respectively subtractNumbers(), multiplyNumbers() and divideNumbers(). After the methods are created and the if statements we should display the result of the operation to the console.
if(operator.equals("+")){
result = addNumbers(num1, num2);
}
if(operator.equals("-")){
result = subtractNumbers(num1, num2);
}
if(operator.equals("*")){
result = multiplyNumbers(num1, num2);
}
if(operator.equals("/")){
result = divideNumbers(num1, num2);
}
System.out.println(result):
}
static double addNumbers(double num1, double num2) {
return num1 + num2;
}
static double subtractNumbers(double num1, double num2){
return num1 - num2;
}
static double multiplyNumbers(double num1, double num2){
return num1 * num2;
}
static double divideNumbers(double num1, double num2){
return num1 / num2;
}
}
Build and run the application
At this point we can run the application to test that it works. Trying out each operation +, -, * and /.
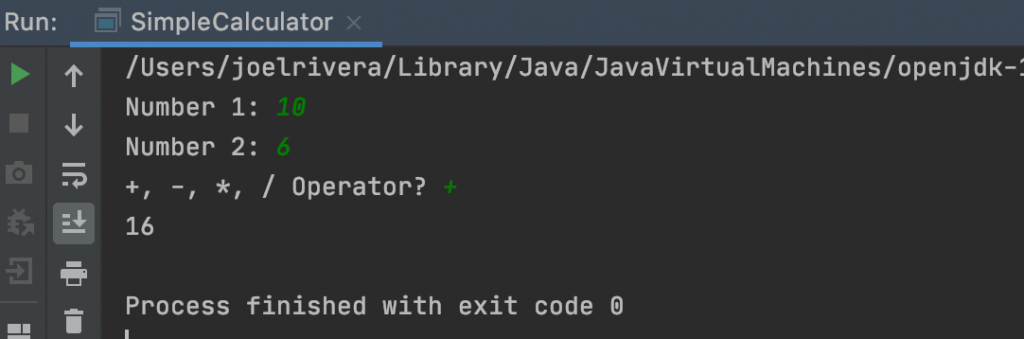
Garbage in garbage out
We are humans, and we make mistakes. Users as well. Sometimes we hit the wrong key when typing. Sometimes a user is deliberate and purposedly will try to sabotage a program by giving it the wrong data. That is why we need to validate. If we don’t, a program can easily crash as the underline OS won’t know what to do. One of the most used methods specifically when validating data that is input in by the user is the try and catch Exceptions that are common predetermined exceptions and errors in the Java programming language.
Let’s place the number requests inside the try. If there is an exception because let’s say the user decides to enter a string instead of an actual number inside the variables the application will catch the exception and store it in the Exception object named e. If an exception is found we must dispose of the garbage that was stored in the variable. We do this by setting the input stream to expect the next entry using the next() method. We will print to the screen that an invalid input has been entered and in addition, it will print the Java util exception stored in the Exception object e.
Last but not least we want the user to reenter the data until he/she enters numerical values. Since we know this needs to be executed at least once. We shall use a do while loop. A do while loop in Java checks its condition at the end of the loop after the loop has been executed. Our condition shall be a boolean variable that we will call flag. We shall place the ‘flag = false;’ once it has completed the storing of the variables, but if an exception is found we shall set the ‘flag = true;’. So at the end of our loop if the flag is set to true, which would mean an exception was caught then it shall repeat the process.
boolean flag;
/* Scanner class is used to collect user input */
Scanner input = new Scanner (System.in);
do try {
System.out.print("Number 1: ");
num1 = input.nextDouble();
System.out.print("Number 2: ");
num2 = input.nextDouble();
flag = false;
}catch(Exception e) {
flag = true;
input.next(); // next() method must be called in the catch block to dispose of the user’s invalid input
System.out.println("Invalid input: " + e);
} while(flag); // Will repeat until until valid numbers stored in variables
Switch case
Previously we used simple if statements in order to determine which operator was entered and therefore which operation will be executed. While yes it does work, it’s not really efficient. Since we are only using the operator as to determine which operation to execute we should use a Switch instead of using multiple ifs. In a switch, we evaluate by using the expression which is compared with the values of each case. In this application, we evaluate the operator which should be only these options +, – , * or /. Anything else entered would be an invalid input. So we place the case statement to evaluate the operator, the default option specifies some code to run if there is no case match. In our case we print out to the screen an invalid input was entered.
As when we verified to see if the user entered proper numbers, we shall have the program repeat the request until the user enters a valid operator. So as before we will use a do while loop. We can reuse the flag variable so long as we remember to to place it to false in the beginning of the do while loop and only change it to true if an invalid input was entered, in our default option.
do {
System.out.print("+, -, *, / Operator? ");
operator = input.next();
//reset the flag to false
flag = false;
switch (operator) {
case "+" -> result = addNumbers(num1, num2);
case "-" -> result = subtractNumbers(num1, num2);
case "*" -> result = multiplyNumbers(num1, num2);
case "/" -> result = divideNumbers(num1, num2);
}
default -> {
/* Invalid input entered flag will be set to true*/
flag = true;
System.out.println("Invalid input: Only allowed operators are +, -, *, /");
}
}
}while(flag); // Will repeat until valid operator is entered
Cannot divide by Zero
There is one other thing we need to look for. Thats right as you may or may not know. Dividing any number by zero is an undefined action as it would be a contradiction in mathematics. Remember when multiplying any number by zero it is zero. Therefore trying to determine what number multiplied by zero would give you that number is impossible.
Ok, I hope I haven’t confused you, as I have been told since of learning this rule “you just can’t divide any number by zero”. So we shall make sure to not force the program to divide by zero, by making sure if the user wants to divide and if they entered a 0 in the second number then we shall send out an error message. If you notice in my example below I print out the following string “Arithmetic error: Cannot divide by “. Because I always return the value of result. If no result was calculated and it was initiliazed at the beginning of the application with the value of 0. The message that the user will see on his/her screen is the following “Arithmetic error: Cannot divide by 0”. If the second entered numbered is anything but zero, then it will execute the divideNumbers() method and return the result of equation.
case "/" -> {
if(num2 == 0) {
System.out.print("Arithmetic error: Cannot divide by ");
break; // Break in order to prevent error
}
result = divideNumbers(num1, num2);
}
One last thing
If we print the double variable ‘result’ at the end of our application it will always display the result with a decimal point. This is true even when we have a whole number at the end of the equation. This is so, because in Java when a floating-point value is printed it forces the floating-point location to appear. In order to display a whole number without a decimal point, we can do a simple equation. If the double result is subtracted by the integer converted result equals zero then we know there is no decimal point and it is a whole number. I used long as the datatype because we are using a double and a long integer allows for bigger integers to be saved in memory.
/* If result is a whole number display as whole and not with a decimal point else just display result */
if(result - (long)result == 0) System.out.println((long) result);
else System.out.print(result);
}
The Final Product
Now we have a completed program and should be able to run it as much as we like.
import java.util.Scanner;
public class SimpleCalculator {
public static void main(String[] args){
double num1 = 0, num2 = 0, result = 0;
String operator;
boolean flag;
/* Scanner class is used to collect user input */
Scanner input = new Scanner (System.in);
do try {
System.out.print("Number 1: ");
num1 = input.nextDouble();
System.out.print("Number 2: ");
num2 = input.nextDouble();
flag = false;
}catch(Exception e) {
flag = true;
input.next(); // next() method must be called in the catch block to dispose of the user’s invalid input
System.out.println("Invalid input: " + e);
} while(flag); // Will repeat until until valid numbers stored in variables
do {
System.out.print("+, -, *, / Operator? ");
operator = input.next();
//reset the flag to false
flag = false;
switch (operator) {
case "+" -> result = addNumbers(num1, num2);
case "-" -> result = subtractNumbers(num1, num2);
case "*" -> result = multiplyNumbers(num1, num2);
case "/" -> {
if(num2 == 0) {
System.out.print("Arithmetic error: Cannot divide by ");
break; // Break in order to prevent error
}
result = divideNumbers(num1, num2);
}
default -> {
/* Invalid input entered flag will be set to true*/
flag = true;
System.out.println("Invalid input: Only allowed operators are +, -, *, /");
}
}
}while(flag); // Will repeat until valid operator is entered
/* If result is a whole number display as whole and not with a decimal point else just display result */
if(result - (long)result == 0) System.out.println((long) result);
else System.out.print(result);
}
static double addNumbers(double num1, double num2) {
return num1 + num2;
}
static double subtractNumbers(double num1, double num2){
return num1 - num2;
}
static double multiplyNumbers(double num1, double num2){
return num1 * num2;
}
static double divideNumbers(double num1, double num2){
return num1 / num2;
}
}