A primitive data type specifies the size and type of variable values, and it has no additional methods. There are eight primitive data types in Java they are the following: byte
, short
, int
, long
, float
, double
, boolean
and char
.
Below you will find a brief explanation of each primitive data types along with example Java programs using the primitive data types.
Byte
The byte
data type can store whole numbers from -128 to 127. It’s default value is 0. It can be used as an integer in order to save memory. Although in this day and age memory is not necessarily a scarce commodity.
public class JustOneByte { public static void main(String[] args){ // A byte is just a small integer that can store value of -128 through 127 // Declaring a variable aByte byte aByte; // Storing the value of 33 aByte = 33; // Display the value in the variable aByte System.out.println(aByte); } }

Short
The short
data type is similar to byte, it holds a numerical integer value. It’s limitation in memory allows it to store whole numbers from -32768 to 32767.
public class ShortList { public static void main(String[] args){ // numberA is a short variable with the value of 14 short numberA = 14; // numberB is a short variable with the value of 156 short numberB = 156; // numberC is a short variable with the value of 842 short numberC = 842; // Now lets list the short list of numbers System.out.println(numberA); System.out.println(numberB); System.out.println(numberC); } }

Integer
The int
data type can store whole numbers from -2147483648 to 2147483647. For the most part int is usually the most used data type when creating whole integer numbers.
public class AddNumbers { public static void main(String[] args){ // firstNumber is a integer variable and its initilized with the value of 3479660 int firstNumber = 3479660; // secondNumber is a integer variable and its initilized with the value of 512499 int secondNumber = 512499; // Now let's add the numbers and store them in a third integer variable result int result = firstNumber + secondNumber; // Finally lets display the result System.out.println(result); } }

Long
The long
data type can store whole numbers from -9223372036854775808 to 9223372036854775807. This is used when int is not large enough to store the value. The long data type should end the value with an “L”.
public class DistanceFromSun { public static void main(String[] args){ // Average distance of Earth from the sun is 92,955,807 miles // milesFromSun is a Long variable long milesFromSun = 92955807L; System.out.print("The average distance of miles between the Earth and the Sun is: "); System.out.print(milesFromSun); } }

Float
The float
data type can store fractional numbers from 3.4e−038 to 3.4e+038. Note that you should end the value with an “f”. The precision of a floating-point value indicates how many digits the value can have after the decimal point. The precision of float
is only six or seven decimal digits.
public class CircumferenceCircle{ public static void main(String[] args){ // Circumference is a floating point variable it has been initialized with 0.0 float circumference = 0.0f; // pi 3.141592 float pi = 3.141592f; // Since we are calculating the circumference of a circle int radius = 3; // Calculate the circumference of the circle when it has a radius of 3 inches circumference = 2 * pi * radius; System.out.println("Circumference of a circle when its radius is 3 inches is: " + circumference + "in"); } }

Double
The double
data type can store fractional numbers from 1.7e−308 to 1.7e+308. Note that you should end the value with a “d”. The double
variables have a precision of about 15 digits or double the float data type. Therefore it is safer to use double
for most calculations.
public class CalculateSlope{ public static void main(String[] args){ // slope is a double floating point variable double slope = 0.000d; // the first point is located (1,4) float x1 = 1.0f; float y1 = 4.0f; // the second point is located (8,7) float x2 = 8.0f; float y2 = 7.0f; // Calculate slope by using the slope equation (y2 - y1) / (x2 - x1) slope = (y2 - y1) / (x2 - x1); System.out.println("Calculate slope m = (y2 - y1) / (x2 - x1)"); System.out.println("Calculate slope m = (7 - 4) / (8 - 1)"); System.out.println("Calculate slope m = " + slope); } }

Boolean
A boolean data type is declared with the boolean
keyword and can only be true
or false
.
public class BooleanExample{ public static void main(String[] args){ // b1 is a boolean variable set to true boolean b1 = true; // b2 is a boolean variable set to false boolean b2 = false; System.out.println("true and true = " + (b1 && true)); System.out.println("true and false = " + (b1 && b2)); System.out.println("false and false = " + (false && b2)); System.out.println("true or true = " + (b1 || true)); System.out.println("true or false = " + (b1 || b2)); System.out.println("false or false = " + (false || b2)); System.out.println("Not true = " + !(b1)); System.out.println("Not false = " + !(b2)); } }
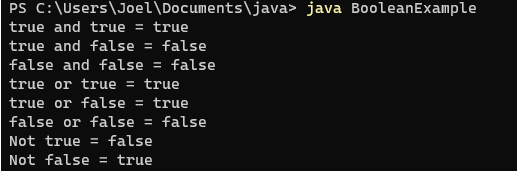
Char
The char
data type is used to store a single character. The character must be surrounded by single quotes, like ‘A’ or ‘a’.
public class CharExample { public static void main(String[] args) { // char1 is a char variable char char1=65; // char2 is a char variable char char2=97; // Display the values of the char variables System.out.println("char1: "+char1); System.out.println("char2: "+char2); // char1 is assigned the value of a Capital B char1 = 'B'; // char2 is assigned the value of a Lowercase b char2 = 'b'; // Display current value of the variables System.out.println("char1 is now: "+char1); System.out.println("char2 is now: "+char2); // Display numerical value of the variables System.out.println("char1 as a number: " + (int)char1); System.out.println("char2 as a number: " + (int)char2); } }
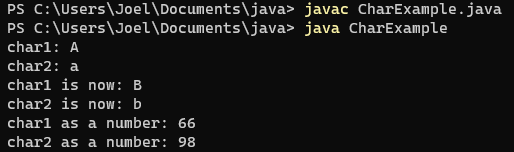